iOS: Creating a Spin Animation using Transitions
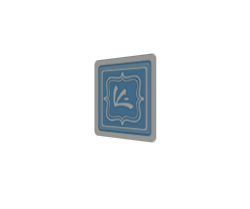
Animations are one of the cornerstones of the iOS platform. Animations can add polish and visual interest to any application.
iOS comes with many default animations and effects. An animation between two views is referred to as a transition.
On a recent project, we needed to create an animation of a card to make it appear spinning. Creating this effect from scratch would involve a fair amount of code, but leveraging transitions made it easy.
Here’s the code that does the magic:
- (void) spinCard: (int) rotations { if (rotations == 0) return; [UIView transitionWithView: card duration: SPIN_DURATION / 2 options: UIViewAnimationOptionTransitionFlipFromLeft + UIViewAnimationOptionCurveLinear animations: ^{ cardFront.hidden = YES; cardBack.hidden = NO; } completion: ^(BOOL finished) { [UIView transitionWithView: card duration: SPIN_DURATION / 2 options: UIViewAnimationOptionTransitionFlipFromLeft + UIViewAnimationOptionCurveLinear animations: ^{ cardBack.hidden = YES; cardFront.hidden = NO; } completion: ^(BOOL finished) { [self spinCard: rotations - 1]; }]; }]; }
What’s going on here? Well, we have a recursive function that leverages the UIViewAnimationOptionTransitionFlipFromLeft transition to flip the card twice, effectively doing a 360° spin. We then decrement the rotation counter and spin again until we get to 0.
Note that using this technique you don’t have quite as much control with respect to the options for the rotation effect as compared with a custom implementation, but as you can see, very little code is needed. It worked great for the effect we needed, and I hope this can help someone else out, too.
If you’d like to clone the example project I created, you can find it in Github here: https://github.com/GrioSF/spincard
1 Comment