Objective-C…it’s not you…it’s Swift
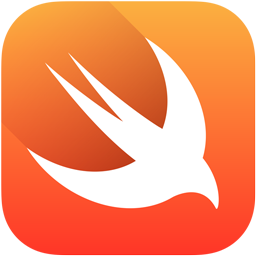
At the lastest Apple WWDC conference, Apple decided to suprise it’s developers with introducing a brand new language called Swift which will be used going forward in development all Mac and iOS applications. The good news for all Apple developers is that it is totally integratable with all existing Objective-C code. Another great positive for developers is that it also runs on the current version of iOS, iOS-7. Developers will still need to wait for Xcode-6 to come out of Beta before they can submit full Swift apps, but they will not require everyone to be running the latest iOS.
Some of the possitives to come with the release of Swift are :
-Infered Typing
-No more Semi-colons
-Interactive Playgrounds
-Generics
-EMOJI Variable names!!!
-No more.h and .m files
When defining a variable in Objective-C, for example a string type, you would write it as follows:
[code language=”javascript”]NSString *string = @"This is a String";[/code]
Now with Swift you no longer have to specifically state the type when declaring the variable. The exact same line of code translates into:
[code language=”javascript”]var string = "This is a String"[/code]
Firstly you will notice “var” instead of “NSString”. Swift can detect automatically what kind of variable type this is and will supply the relevant methods that go with it’s type such as string.lowercase() or string.substringFromIndex(index: Int). Another thing to notice is the missing *. Swift now avoids giving you direct access to pointers. This is in an effort to reduce developer errors for knowing when the it is correct to use pointers. They are still accessible under the hood though. Lastly is the missing Semi-colon. YAY!!!
One thing to note also is that all “var” variable definitions are mutable. To define a constant you simply use the “let” to define your variable.
Another awesome feature that has been brought in with the introduction of Swift is Playgrounds.
Playgrounds is a way of writing code and instantly seeing the results. It can display print outs in a console, draw variables on a graph and even display views. This means it is a great way to test out new algorithms, play with new API’s and even design and watch animated scenes using SpriteKit. I also found it a great way to get my feet wet when I first started developing with Swift.
Generics is major part of the language. Most of the language itself is built with generic code for example 2 very common datastructures, arrays and dictionaries, are totally generic collections.
[code language=”javascript”]
//Non-Generic function
func swapTwoInts(inout a: Int, inout b: Int) {
let temporaryA = a
a = b
b = temporaryA
}
//Generic function
func swapTwoValues<T>(inout a: T, inout b: T) {
let temporaryA = a
a = b
b = temporaryA
}
[/code]
This is an example taken from the official Apple Swift Programming Language book. It shows 2 simple functions for switching 2 variables. In the generic function, Swift knows that “T” is just a placeholder. It doesnt look for type “T”. As “T” is given for the variable types of both input parameters, Swift knows to only allow this method be run with 2 variables of the same type.
One of the more fun features of Swift, that is totally unnessary but totally necessary at the same time, is it’s ability to use any character as a variable names, class names and function names. Including EMOJI symbols!!!!
As ludacris as this code may look, it is perfectly acceptible to write this in your Swift project and have it compile and run without any issues what so ever. I have yet to find a useful implementation using EMOJI’s, but it certainly makes it a little more fun!
Swift now releases all Apple developers from the need to create .h files alongside your .m file. This means that when defining variables and methods, they are always default to public. Though since it’s original release back in June, Apple have since stated that they will be introducing access control mechanisms to give developers more control.
Integrating Swift with preexisting Objective-C code is quite straightforward. I have been working with SpriteKit a lot recently and came across a handy class called SKButton, which is an extension of SKSpriteNode. It is convenient way to create buttons within your game and called custom actions for each button press and is written entirely in Objective-C. When you introduce a .m file into a Swift project you will get prompted to create a bridging header. CLICK YES!!! Inside this bridging header, you can define all the files your swift code can have access to. When using Swift code with existing Objective-C code, all you have to do is add
[code language=”javascript”]#YourProjectName#-Swift.h[/code]
to whatever .m file needs access to your Swift objects. This .h file is created automatically upon creation of your project.
Links to handy resources:
https://developer.apple.com/library/prerelease/ios/documentation/Swift/Conceptual/Swift_Programming_Language/
http://kpbp.github.io/swiftcheatsheet/
http://www.swifttoolbox.io