Chrome Extension Basics
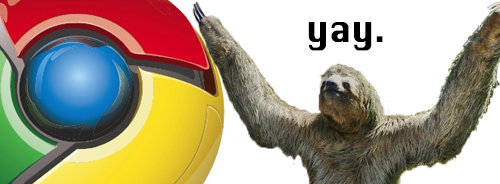
I use Chrome extensions all the time and decided it was time to figure out how to make my own. I found it to be incredibly easy and I’d like to share with you some of the basics, as well as an example of an extension I made. Let’s get started!
A Chrome whuuuu?
If you’ve ever been browsing the internet and thought, “I wish I could enhance my online shopping experience by being able to apply discounts at the touch of a button.” OR “Wow, this web page is great but it would be even better upside-down!”…
Good news: There are Chrome extensions to do both of those things. Extensions are small software programs written with HTML, CSS and JavaScript that enhance the out of the box functionality of Google Chrome. They also come in many different flavors. From helpful, production boosting extensions to the downright absurd, there’s an extension for just about anything.
Extensions are able to:
- Make cross-domain AJAX requests and use 3rd party web services
- Use local storage – up to a 5MB limit
- Create UI elements outside of the rendered web page
- Communicate with other extensions
- Run background scripts
- Access many special purpose APIs that normal webpages can’t
If you can’t find what you’re looking for, build it yourself. You can have a bare bones extension installed and running in no time at all.
Components
All extensions require a manifest.json file. Quite simply, it’s a JSON formatted file that contains key-value pairs of settings and pointers to extension content. Here’s what a manifest might look like with the absolute minimum required information:
{
"manifest_version": 2,
"name": "The greatest extension ever",
"version": "1.0",
}
That’s it. That’s all you need – you can open up chrome and load your extension (more info on that later). What will it do? Nothing at all… so let’s make it do something.
Some other common components include:
- Background Page
The Background Page can be thought of as the main logic of the extension. A background page can be made up solely of JavaScript files and requires no HTML markup at all. It runs in the background ( who would have guessed? ) and can either be persistent or not. By setting the background page “persistent” value to false, it becomes an ‘Event Page’. Event Pages can listen for events and are only loaded when needed.
- Browser Action *OR* Page Action
You can pick one or the other… or neither. These components are usually used as a UI integration point. If you’ve ever installed an extension, you may have noticed an icon appear in your browser. If the icon appeared in the address bar, then the extension contained a Page Action. If the icon appeared directly beside the address bar – that’s a browser action. Page Actions contain page specific functionality, whereas Browser Actions can be used to create pop-ups that contain an interface for the user to interact with the extension.
- Content Scripts
Content Scripts are JavaScript files that can be used to read and manipulate the content of a website that a user visits. Perhaps you hate the background color of a website – use a content script to change it to something more to your liking. Content Scripts are limited in their access to the chrome.* APIs.
These are just a few components that you can use to build an extension. All of these components need to be registered within your manifest.json file. You can set up your project architecture however you want, but the manifest.json must be in the root directory of your project. Any files you want to add (HTML, CSS, Javascript) should be referenced in the manifest according to your project layout.
Let’s Build Something
Sometime when I’m browsing the internet, I feel like I need to be inspired. I want to be able to click an icon and get the inspiration I’m looking for. Basically, I want to inject some content into any page I’m on and see it when I click on a button.
Here’s what I need:
- Browser Action – Simply used to show an icon and act as a button to do something when I click it.
- Background Page – A bit of script that runs in the background that listens for a click on the browser action and run some script within the context of my current tab (have to register tab permissions in manifest.json)
- Script to be run when the Background Page catches a click event on the Browser Action. (similar to Content Script – but I’m not registering it as such because I only want it to run when a click event is caught)
- Assets within my extension that I want to be accessed from the current page I’m on. ( I’ll need to set up a ‘web_accessable_resources’ property in the manifest )
Sweet – That’s all I need. Here’s what my manifest.json looks like:
{
"manifest_version": 2,
"name": "Sloth Rain",
"version": "1.0",
"browser_action": {
"default_icon": "resources/icons/dollar-icon.png"
},
"background": {
"scripts": ["background/background.js"]
},
"permissions": ["tabs", "http://*/*", "https://*/*"],
"web_accessible_resources": ["inject/assets/*"]
}
So all I’m doing with my Browser Action is setting an icon. When I install the extension, that icon will be displayed to the right of the address bar. I said I wanted to do something when I click on the icon… that’s what the Background Page is for. It uses the chrome.* APIs to listen for a click. Here’s what the Background Page looks like:
chrome.browserAction.onClicked.addListener(function(){
chrome.tabs.executeScript(null, {file: "inject/content.js"});
});
All it is doing is listening for a click event to occur on the Browser Action and then it’s running the script I declared within the context of the current tab. content.js is doing a lot of the heavy lifting and might be a bit much to show here… but don’t worry, I’ll provide a link to download the extension which you can install / tinker and tweak to your heart’s desire. Basically, it creates some img
elements and appends them to the DOM and animates them. After 10 seconds all the newly created elements are removed from the DOM to allow for a normal uninspiring browsing experience. The src attributes of the created img elements point to files included with the extension, which is why "web_accessible_resources": ["inject/assets/*"]
is declared in the manifest file above.
Here’s a screen shot of the end result:
Awesome – That guy looks like he’s living the life.
Installation & Hosting
You can host or install extensions from the Chrome Web Store: https://chrome.google.com/webstore/category/extensions
To host in the Web Store you’ll need to set up an account and pay a one time registration fee. After submitting your extension it will undergo an automated review process and in most cases will be available almost immediately.
You can also personally host your extension. If you choose to host your extension, you are responsible for packaging it as a .crx file. You are also required to set up an auto-update manifest. More information on privately hosting your extensions: https://developer.chrome.com/extensions/hosting
Perhaps you’re developing an extension and want to try it out without all the hassle of packaging and hosting… Maybe you’re just making an extension for yourself and have no plans on sharing with the rest of the world. You can easily install an extension directly in Chrome. You can try it out by downloading and installing the extension I created.
- Get the code here: https://github.com/DevinJohnson/SlothExtension
- In the address bar, navigate to chrome://extensions/
- Navigate to and select the extension’s root directory ( the folder containing manifest.json )
You can also update, disable and delete your installed extensions from chrome://extensions/
For more detailed information on how to get started or information about specific components, go here: https://developer.chrome.com/extensions
Be inspired and go build something. Happy Coding!
1 Comment