Updating React Native Firebase from v5.6.0 to v14.2.0
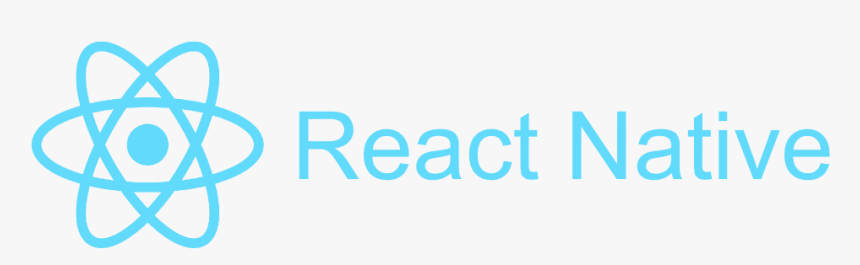
The software developer’s job is not done once an application is created. As device operating systems are updated, apps must be updated as well to remain compatible. To demonstrate a typical app upgrade, this post goes through an upgrade I recently performed on a React Native mobile app.
Upgrading the React Native Firebase Library
Our React Native mobile application currently uses the react-native-firebase package to receive and display notifications that are sent through our Google Firebase project. However, Google Play Store recently released required updates for Android 13, and our version of react-native-firebase, version 5.6.0, was rendered incompatible with devices using Android’s new target SDK. We, therefore, needed to upgrade from version 5.6.0 to the newest version, 14.2.0.
To migrate to version 14.2.0, I had to take the following steps:
Step 1: Remove react-native-firebase:5.6.0 package.json
“react-native-firebase”: “^5.6.0”
Step 2: Remove react-native-firebase from Android
1. Remove react-native-firebase from the android/settings.gradle
include ‘:react-native-firebase’
project(‘:react-native-firebase’).projectDir = new File(rootProject.projectDir, ‘../node_modules/react-native-firebase/android’)
2. Remove any references to the io.invertase.firebase.messaging class from the AndroidManifest.xml, as they are no longer needed
<receiver android:name=”io.invertase.firebase.notifications.RNFirebaseNotificationReceiver”/>
<receiver android:enabled=”true” android:exported=”true” android:name=”io.invertase.firebase.notifications.RNFirebaseNotificationsRebootReceiver”>
<intent-filter>
<action android:name=”android.intent.action.BOOT_COMPLETED”/>
<action android:name=”android.intent.action.QUICKBOOT_POWERON”/>
<action android:name=”com.htc.intent.action.QUICKBOOT_POWERON”/>
<category android:name=”android.intent.category.DEFAULT” />
</intent-filter>
</receiver>
<service android:name=”io.invertase.firebase.messaging.RNFirebaseMessagingService” android:exported=”true”>
<intent-filter>
<action android:name=”com.google.firebase.MESSAGING_EVENT” />
</intent-filter>
</service>
<service android:name=”io.invertase.firebase.messaging.RNFirebaseInstanceIdService” android:exported=”true”>
<intent-filter>
<action android:name=”com.google.firebase.INSTANCE_ID_EVENT”/>
</intent-filter>
</service>
<service android:name=”io.invertase.firebase.messaging.RNFirebaseBackgroundMessagingService” />
3. Remove references to react-native-firebase and com.google.firebase from android/app/build.gradle
implementation project(‘:react-native-firebase’)
implementation ‘com.google.firebase:firebase-core:16.0.9’
implementation ‘com.google.firebase:firebase-inappmessaging-display:17.2.0’
implementation ‘com.google.firebase:firebase-messaging:18.0.0’
4. Remove the react-native-firebase packages from android/app/src/main/java/com/<application name>/mobile/MainApplication.java
import io.invertase.firebase.RNFirebasePackage;
import io.invertase.firebase.analytics.RNFirebaseAnalyticsPackage;
import io.invertase.firebase.messaging.RNFirebaseMessagingPackage;
import io.invertase.firebase.notifications.RNFirebaseNotificationsPackage;
new RNFirebasePackage(),
new RNFirebaseAnalyticsPackage(),
new RNFirebaseMessagingPackage(),
new RNFirebaseNotificationsPackage(),
5. Clean gradle in preparation for a new installation by running:
cd android
./gradlew clean
Step 3: Remove react-native-firebase from iOS
1. Remove RNFirebase, Firebase/Core, Firebase/Messaging/ Firebase/InAppMessaging pods from ios/Podfile
pod ‘Firebase/Core’
pod ‘Firebase/Messaging’
pod ‘Firebase/InAppMessaging’
pod ‘RNFirebase’, :path => ‘../node_modules/react-native-firebase/ios’
2. Remove the pods from the project by running:
cd ios
rm -rf Podfile.lock
pod install
Step 4: Install the New Version of @react-native-firebase/app and the Additional Modules
1. Many of the react-native-firebase modules that were once included in the core module have now been moved to separate modules.
yarn add @react-native-firebase/app@14.12.0
yarn add @react-native-firebase/analytics@14.12.0
yarn add @react-native-firebase/messaging@14.12.0
yarn add @notifee/react-native@5.6.0
Step 5: Add the @react-native-firebase/app and the Additional Packages
1. Add @react-native-firebase/app and the additional packages to android/settings.gradle
include ‘:@react-native-firebase_app’
project(‘:@react-native-firebase_app’).projectDir = new File(settingsDir, ‘../node_modules/@react-native-firebase/app/android’)
include ‘:@react-native-firebase_analytics’
project(‘:@react-native-firebase_analytics’).projectDir = new File(settingsDir, ‘../node_modules/@react-native-firebase/analytics/android’)
include ‘:@react-native-firebase_messaging’
project(‘:@react-native-firebase_messaging’).projectDir = new File(settingsDir, ‘../node_modules/@react-native-firebase/messaging/android’)
include ‘:@notifee_react-native’
project(‘:@notifee_react-native’).projectDir = new File(settingsDir, ‘../node_modules/@notifee/react-native/android’)
2. Add @react-native-firebase/app and the additional packages to android/app/build.gradle
implementation project(‘:@react-native-firebase_app’)
implementation project(‘:@react-native-firebase_analytics’)
implementation project(‘:@react-native-firebase_messaging’)
implementation project(‘:@notifee_react-native’)
3. Add @react-native-firebase/app and the additional packages to android/app/src/main/java/com/rivals/mobile/MainApplication.java
import io.invertase.firebase.app.ReactNativeFirebaseAppPackage;
import io.invertase.firebase.analytics.ReactNativeFirebaseAnalyticsPackage;
import io.invertase.firebase.messaging.ReactNativeFirebaseMessagingPackage;
import io.invertase.notifee.NotifeePackage;
new ReactNativeFirebaseAppPackage(),
new ReactNativeFirebaseAnalyticsPackage(),
new ReactNativeFirebaseMessagingPackage(),
new NotifeePackage(),
Step 6: Replace the Package Import References with @react-native-firebase/app in the Project Code Throughout the Application
import firebase from ‘react-native-firebase’;
import firebase from ‘@react-native-firebase/app’;
1. Additional modules can be imported in the src/App.tsx. Once this is done, they can be referenced through the Firebase/app instance.
2. Note some of the new package api methods have changed such as analytics.setCurrentScreen to analytics.logScreenView
import firebase from ‘@react-native-firebase/app’;
import { analytics } from ‘react-native-firebase’;
import ‘@react-native-firebase/analytics’;
import ‘@react-native-firebase/messaging’;analytics().setCurrentScreen(newTab.routeName);
firebase.analytics().logScreenView({ screen_name: newTab.routeName });
Step 7: Remove react-native-firebase/notifications and Use @notifee/react-native in the Project Code
One of the biggest changes from react-native-firebase 5.6.0 to 14.2.0 is the removal of the notifications package. I chose to use the recommended package @notifee/react-native package for version 14.2.0. This required removing react-native-firebase/notifications and using the @notifee/react-native in the project code.
import firebase, { RNFirebase } from ‘react-native-firebase’;
import { NotificationOpen } from ‘react-native-firebase/notifications’;import firebase from ‘@react-native-firebase/app’;
import { FirebaseMessagingTypes } from ‘@react-native-firebase/messaging’;
import notifee, { AndroidImportance, EventType, Notification, Event } from ‘@notifee/react-native’;private messaging: RNFirebase.messaging.Messaging;
private messaging: FirebaseMessagingTypes.Module;private notifications: RNFirebase.notifications.Notifications;
this.notifications = firebase.notifications();
this.notifications.onNotification(notification => {});
this.messaging.onMessage(messageNotification => {});this.notifications.displayNotification(headsUpNotification);
notifee.displayNotification(headsUpNotification);
notifee.onForegroundEvent(({type:Event)=>{
if(type === EventType.Press){
this.navigateFromNotification(headsUpNotification);
}
})this.notifications.onNotificationOpened(notification =>{});
this.messaging.onNotificationOpenedApp(notification=>{});
this.notifications.removeDeliveredNotification(notification.notificationId);
notifee.cancelNotification(messageId);const channel = new firebase.notifications.Android.Channel(‘stuff-channel’,’Stuff Channel’,firebase.notifications.Android.Importance.Max ).setDescription(‘Notifications about stuff ‘);
this.notifications.android.createChannel(channel);
notifee.createChannel({id: ‘stuff-channel’, name: ‘Stuff Channel’, importance: AndroidImportance.HIGH, description: ‘Notifications about stuff’});
Testing the Upgrade
Once I completed the upgrade, I then attempted to build and run the mobile application on an Android emulator.
Once the app was up and running on my Android emulator, I verified that notifications were working by opening my project’s web browser Firebase console, and composing a test notification to send to my emulator.
Overall, this was a relatively straightforward update. However, not every update goes exactly according to plan. To learn more about some of the challenges associated with updated applications, check out Debugging Difficult Things. To find out how Grio can help you with your app upgrades, contact us today.