FBLoginView === Facebook authentication made easy with SDK 3.x
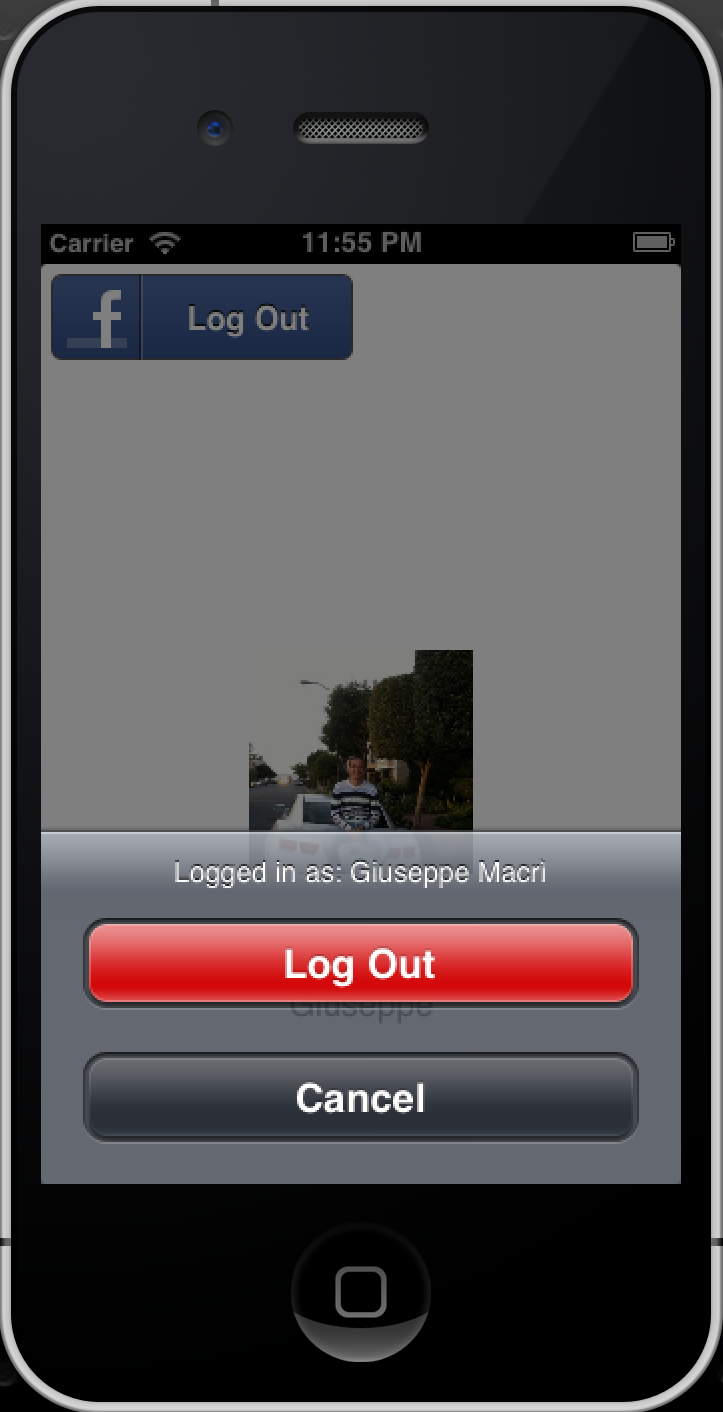
This post will explain how to use a new component within the Facebook SDK iOS; I am talking about FBLoginView.
Facebook SDK for iOS has seen several updates and improvements during the last year. During my first project I was asked to refactor the authentication flow, such as login and logout.
I started creating a class, singleton, to handle Facebook sessions status using the following code:
[code lang=”java”]
//
// Facebook.h
//
// Created by Giuseppe Macri on.
// Copyright (c) 2012 FJ. All rights reserved.
//
#import <Foundation/Foundation.h>
@protocol facebookDelegate
– (void)facebookLoginSuccess;
– (void)facebookLoginFailed;
@end
@interface Facebook : NSObject
@property (nonatomic, weak) id delegate;
+ (id)sharedInstance;
– (void)openSession;
– (void)closeSession;
– (BOOL)checkSession;
@end
//
// Facebook.m
//
// Created by Giuseppe Macri on.
// Copyright (c) 2012 FJ. All rights reserved.
//
#import "Facebook.h"
#import <FacebookSDK/FacebookSDK.h>
@implementation Facebook
+ (id)sharedInstance
{
static dispatch_once_t pred = 0;
__strong static id _instance = nil;
dispatch_once(&pred, ^{
_instance = [[self alloc] init]; // or some other init method
});
return _instance;
}
– (void)sessionStateChanged:(FBSession *)session
state:(FBSessionState) state
error:(NSError *)error
{
switch (state) {
case FBSessionStateOpen: {
FBCacheDescriptor *cacheDescriptor = [FBFriendPickerViewController cacheDescriptor];
[cacheDescriptor prefetchAndCacheForSession:session];
[self.delegate facebookLoginSuccess];
}
break;
case FBSessionStateClosed:
case FBSessionStateClosedLoginFailed:
[FBSession.activeSession closeAndClearTokenInformation];
break;
default:
break;
}
if (error) {
UIAlertView *alertView = [[UIAlertView alloc]
initWithTitle:@"Error"
message:error.localizedDescription
delegate:nil
cancelButtonTitle:@"OK"
otherButtonTitles:nil];
[alertView show];
[self.delegate facebookLoginFailed];
}
}
– (void)openSession
{
[FBSession openActiveSessionWithReadPermissions:nil
allowLoginUI:YES
completionHandler:
^(FBSession *session,
FBSessionState state, NSError *error) {
[self sessionStateChanged:session state:state error:error];
}];
}
– (void)closeSession
{
[FBSession.activeSession closeAndClearTokenInformation];
}
– (BOOL)checkSession
{
if (FBSession.activeSession.state == FBSessionStateCreatedTokenLoaded) {
return YES;
} else {
return NO;
}
}
@end
[/code]
Besides the Facebook class implementation I also had to implement the UI and action controller method in order to call the authentication singleton and perform the login request.
While I was playing around with the new Facebook SDK 3.1 for iOS I noticed the new FBLoginView component.
FBLoginView class offers useful functionalities to support authentication flow logic and UI for both login and logout.
I decided to replace my previous implementation with the FBLoginView using the following code:
[code lang=”java”]
– (void)viewDidLoad
{
[super viewDidLoad];
FBLoginView *loginview = [[FBLoginView alloc] init];
loginview.frame = CGRectOffset(loginview.frame, 5, 5);
loginview.delegate = self;
[self.view addSubview:loginview];
[loginview sizeToFit];
}
#pragma mark – FBLoginViewDelegate
– (void)loginViewShowingLoggedInUser:(FBLoginView *)loginView {
}
– (void)loginViewFetchedUserInfo:(FBLoginView *)loginView
user:(id)user {
self.usernameLabel.text = user.first_name;
self.profilePic.profileID = user.id;
}
– (void)loginViewShowingLoggedOutUser:(FBLoginView *)loginView {
self.profilePic.profileID = nil;
self.usernameLabel.text = nil;
}
[/code]
The above code is everything the developer needs to write; no UI implementation and/or session handlers are required.
The implementation checklist for using FBLoginView is the following:
· Create a FBLoginView instance and add it to the subview controller
· Customize the delegate method to serve an authenticated user
· Customize the delegate method to serve a not authenticated user.
I created a git repository with the entire project with some extra functionality, such as displaying user profile picture. You can find the repo here.
The git project can also be used as a template for your social apps, you only need to update the faceboook app ID.
FBLoginView is a really useful class and very straightforward. It also comes in really handy for prototyping.
I really appreciate all the effort Facebook is putting in with their new SDKs.
Happy Coding!
5 Comments