Introduction To Core Data
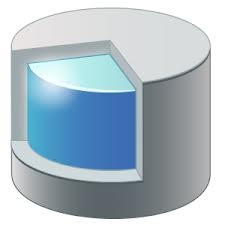
Core Data is a framework provided by Apple that allows developers to design, build and interact with a database, without ever having any direct interaction with SQL.
Recently I completed a project that was entirely based around Core Data. The app needed to be completely dynamic, allowing the user the ability to add and edit new and current functionality through the app itself. Core Data allowed us to interact with database as if it was a collection of objects, thus resulting in a very easy and efficient way to edit the database and structure.
XCode provides a lot of help and guidance when setting up a project that will use CoreData. When starting a new project, make sure to check the option “Use Core Data.” Doing this will result in XCode preloading your project with a .xdatamodel file. This is your database. Also inside your AppDelegate, some properties and functions have been preloaded which correlate with the interaction and maintenance of the database. When opened, you have the option to view the database as a plain list of entities and attributes, or as a graphical representation of your database.
At the bottom of the screen are two options. “Add Entity” and “Add Attribute.” The entities refer to the objects that will be in our database, and the attributes of those objects. Within each entity you must also define how they relate to each other.
Here I have defined a simple database that contains a collection of people. Each “Person” can have multiple “Addresses” and can have multiple “Friends,” each “Address” only has one owner. Core Data takes care of all the unique ID’s that would usually be required if written in SQL.
Once you have defined your database, you next need to have XCode generate the classes for each entity. To do this you simply click on an entity and then select “Create NSManaged Object Subclass” which can be found under the “Editor” tab. This will then create a .h and .m file for your object, it also pre-populates the class with basic functionality for adding and removing objects to the relationships, e.g. add person to the list of friends, add addresses. One thing to note is that every time you update the entities in your model, you must re-generate both files and delete the old ones. ONLY REMOVE REFERENCE AND DON’T MOVE TO TRASH!!!
To interact with the database, we use the properties that have been preloaded into our AppDelegate. “NSManagedObjectContext” is pretty much the top dog as this is what we will use for (almost) all of our interaction with the database. “NSManagedObject” is also important as this is will contain the definition for each of your objects inside your model.
[code lang=”java”]
NSManagedObjectContext *context = [self managedObjectContext];
Person *person = [NSEntityDescription insertNewObjectForEntityForName:@"Person" inManagedObjectContext:context];
person.name = @"Ian";
person.age = @25;
Address *address = [NSEntityDescription insertNewObjectForEntityForName:@"Address" inManagedObjectContext:context];
address.street = @"2nd St";
address.number = @215;
address.owner = person;
[person addAddressesObject:address];
[/code]
This is the code required to populate your database. Get a pointer to the database context and then, using the classes we generated, we can create new entities and populate them with their relevant data types.
[code lang=”java”]
NSError *error;
NSFetchRequest *fetchRequest = [[NSFetchRequest alloc] init];
NSEntityDescription *entity = [NSEntityDescription entityForName:@"Person" inManagedObjectContext:context];
[fetchRequest setEntity:entity];
NSArray *fetchedObjects = [context executeFetchRequest:fetchRequest error:&error];
for(Person *person in fetchedObjects){
NSLog(@"Name : %@, Age : %@",person.name, person.age);
}
[/code]
Extracting objects from the database is just as easy as inserting, all you have to do is create a “NSFetchRequest,” set the entity type you are looking for and it will return all of the objects in the database of that class type. Easy Peasy!
The beauty of Core Data is the easy of use. There is no need to worry about SQL statements. The only hint of SQL is when you want to add a where clause to your “NSFetchRequest.” To do this you just add a “NSPredicate” to your “NSFetchRequest” with the constraints that you provide.
Core Data Rules!!!