Prototyping with P5
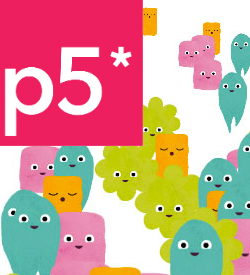
When I wanted to create a proof of a concept project for one of the game ideas I had, I found many tools and gaming frameworks. However, most of them had a steep learning curve which required a significant time investment before the game could be played. Then I discovered P5, a Javascript library inspired by the Processing language, which made it very easy to get started.
So what is Processing?
Processing is a Java-based language that runs on MacOS, Windows, and GNU/Linux platforms. It was created byBen Fry and Casey Reas, who were graduate students at MIT at the time. The language was inspired by BASIC and Logo which were then well known and well used. Ben and Casey’s focus was to use Processing as an educational tool to teach computer science through a visual context as it would be more influential for students getting into programming to make things appear on the screen.
Processing emerged from teaching programming in visual context to a production-quality application development tool for professionals. It is used for data visualization, special effects, 3d printing, and more. It is often used in conjunction with other programming tools and languages such as Arduino, Raspberry Pi, Ruby and Scala. Processing also supports different mods out of the box to develop with Python, JavaScript, Coffee Script, and Android.
Then what is P5?
John Resig, creator of jQuery, helped the Processing team build Processing.js. It executes PDE files within HTML 5 utilizing the Canvas object. It translates Java-based PDE code to JavaScript code using Regex. Due to problems with translating languages, Processing stopped supporting JavaScript and Coffee script modes with the release of Version 3. At that time P5 was created.
Lauren McCarthy created P5 and is a JavaScript library using the P5 API. Being a JavaScript library, it can interact with HTML5 elements as well as sound, webcam, text, and video input while still having a similar IDE structure as Processing.
The power to interact with visual element makes P5 a super tool. It supports physical manipulation, hardware input, image, voice support, multi touch, and 2D/3D graphics with WebGL. P5 also has wide range of 3rd party libraries to handle Geolocation, OCD (Obsessive Camera Direction), audio sequencing, physics, and much more.
Code example
I decided to show prototyping with P5 using simple pong game as an example. If you are not familiar with Pong, it is a 2 dimensional table tennis game.
In P5 there are two main functions: setup() and draw(). The setup() function is executed once when you start the program and draw() loops 60 times per second by default. createCanvas() is another P5 function that takes width and height as parameters to initialize an HTML Canvas element.
[code language=”javascript”]
function setup(){
createCanvas(600,600);
}
function draw(){
}
[/code]
Below I have created the SmallBall class to simulate a moving ball. Its display() function includes P5’s fill function that takes RGB and an optional opacity value to apply color to an object created directly below it. The ellipse() function takes x and y coordinates of the object as well as the width and height to adjust its size and position. The move() function updates the ball’s x and y coordinates each frame byr.
[code language=”javascript”]
function SmallBall() {
this.x = width/2;
this.y = height/2;
this.diameter = 25;
this.velocityX = 2.16;
this.velocityY = 3.72;
this.move = function(){
this.x = this.x + this.velocityX;
this.y = this.y + this.velocityY;
};
this.display = function() {
fill(65,65,65);
ellipse(this.x, this.y, this.diameter, this.diameter);
};
};
[/code]
The Player class is similar to SmallBall class but uses the rect() function which takes x and y coordinates plus width and height values to create a rectangle.
[code language=”javascript”]
rect(this.x, this.y, this.width, this.height);
[/code]
In order to handle keyboard input, P5 provides keyPressed() and keyReleased() functions which listens for key events. Pressing Q and W toggles leftKeyPressed and rightKeyPressed properties respectively and triggers movements in the x coordinate axis of the Player object.
[code language=”javascript”]
function keyPressed() {
if(key == ‘Q’) {
player1.leftKeyPressed = true;
} else if(key == ‘W’) {
player1.rightKeyPressed = true;
}
}
function keyReleased() {
if(key == ‘Q’) {
player1.leftKeyPressed = false;
} else if(key == ‘W’) {
player1.rightKeyPressed = false;
}
}
[/code]
To finish off, I have added two players in the setup function and executed its actions in draw function. Below is what the final prototype looks like.
Here are links to the demo, full code, and detailed documentation for Processing and P5:
Demo: Pong Demo
Demo Source Code: Pong Demo Repo
More information about P5: http://p5js.org/
More information about Processing: https://processing.org/
I hope you consider using P5 to quickly prototype your next project weather it is a web ui component, a game, or any type of digital art!