Using HAML with Ruby on Rails
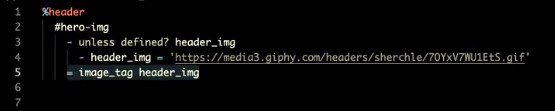
Big Picture
The ultimate goal of any webpage is to display useful information to a user. What the user sees on the page is commonly referred to as the view layer. Over the years various methods of delivering views have been developed.
In the beginning, there was HTML. But pure HTML (markup) had a significant drawback in that it is static, meaning that the markup, once written, was exactly the same for all users all the time.
Views needed to evolve to become more dynamic. We could alter markup already rendered to the user with Javascript, but this can be cumbersome and generally requires additional requests which leads to longer load times. Better to render a view from the onset which contains customizations for each user? The answer in the Ruby realm came via Embedded Ruby HTML or ERB. This gave us the ability to inject Ruby code directly into our markup prior to a page being rendered to the user.
Example:
HTML
ERB
HAML
While slightly longer, the benefit of ERB is that we can deliver a customized experience that is dynamic for each user. This represents the core benefit of ERB over HTML.
The last example is HAML, which we’ll be discussing today. This takes this concept of embedded Ruby in HTML a step further by reducing the amount of code required to generate these views.
This initial example very basic, and it may not seem like there is much difference. The advantages of HAML over ERB will become apparent as the size and complexity of your views expand over time.
Rendering
When you render your application, it creates the view, or the screen that is presented to the user. Views are typically rendered from Rails controllers. By default, Rails will render the view that is found in:
- The folder matching the Controller name
- The filename matching the control action
For example, UsersController#show will render the view app/view/users/show.html.erb.
While this is the default setting of Rails, it can be overwritten as needed. Different views can also be defined based on certain values within the rendering sequence or a specific user state.
Layouts
In Rails, all rendered views have a pre-applied layout. This allows the standardized templates and logic to be easily shared by many individual views.
The default layout in Rails is usually ‘application.html.haml’ or ‘application.html.erb’.
Partials
Partial templates, also known as partials, allow you to break the rendering template into smaller, more manageable sections. Partials also allow you to extract pieces of code from separate files so that, rather than retyping the code in each new location, it can be quickly referenced with a single line of code.
Partials are typically rendered from within another view. Partial filenames always start with an underscore: ie: _table.html.haml. However, the underscore is omitted if the partial is not in the same folder as the originating view where it is being called, as seen here:
Locals
When rendering a partial, it is best to always explicitly pass the variables needed via the :locals hash. This makes your partial more atomic as it will not consume @instance_variables which may or may not be in scope.
Items in the :locals hash may be nested, and the hashes will be merged just like a Ruby arguments hash:
HAML Introduction
HAML, also known as HTML abstraction markup language, is a templating language that was designed to make the HTML cleaner while providing more dynamic content in HTML. On Rivals, we use HAML to mark up our embedded Ruby views.
Why HAML
Some of the greatest benefits of HAML include:
- Clean(er) code
- Enforces correct white spacing by default
- Less typing seems like a plus
- Promotes consistent code style
Other advantages of HAML are discussed below.
White Space Sensitive
White space refers to the spaces, tabs, and line breaks within a code block that make the code easily readable. As mentioned above, HAML enforces correct white spacing by default.
For example, you can see the delineation between HTML and HAML code here:
As you can see, the HAML code on the right uses breaks to make the code easier to understand. For example, If a section of the code is a child of its parent, it is denoted by being tabbed under the parent.
Basic Syntax
Some of the basic syntax that you can use in HAML include:
- Use “=” to evaluate and display Ruby code.
- Use “-“ to evaluate and NOT display Ruby code.
- Use “%” to create a tag of a particular tag. This allows you to create any standard element you would have created in HTML by simply proceeding it with the “%”. For example:
- %strong
- %br
- %body
For example, in the code below, we are defining the header image that will be used:
As you can see, the third and fourth lines are proceeded by an “-“, which means that the information will be evaluated but not displayed on the user interface (UI). However, the final line is proceeded by an “=”, which means that the header_img will be displayed on the UI.
Auto Div
Div is the most common HTML tag in most projects. HAML uses this to assist in code creation. If you fail to use the proper syntax, such as failing to use the percent sign in the previous example, HAML creates an automatic div for the tag.
As you can see above, an automatic div has been implied to the second line of this code. Instead of using %div.center, we used .center and the div was automatically implied.
Classes & IDs
HAML also offers an easy solution for denoting classes and IDs:
- If you begin a HAML line with a “.” or if you have a “.” anywhere in the declaration, anything that follows will be applied as a class name.
- If you begin a HAML line with a “#” anything that follows will be applied as an ID.
Classes and IDs can be chained together in any order, mixed and matched, passed as a hash, or passed as a variable reference that contains a list of classes and IDs.
Filters
Filters allow you to pass syntax from another language which will be compiled into output. This means that at any point in the HAML code, you can stop writing HAML and instead start writing in a different language. In the example below, this is denoted by the “:Javascript” at the beginning of the code.
This is a beneficial feature because it allows you to utilize the benefits of Javascript and other languages within your HAML view.
Object Class Notation
You can further define class and ID through HAML using object class notation to create dynamic classes and IDs.
For example, in the code below, we have identified the user as “crazy.”
Within our HAML view, we can then add square brackets on the end of the user profile and HAML will generate, from that single object, both a class and ID on a dynamic basis. The second argument is an optional perpended string value for the class or ID.
If our user is user 15, then the resulting div has a class of “greeting_crazy_user,” and an ID of “greeting_crazy_user,” along with the specific element it is evaluating (in this case, the number 15):
Object class notation comes in handy if you want multiple classes and IDs that are dynamic.
Final Thoughts
While HAML is quickly being replaced by newer languages, such as React, it is nonetheless still a beneficial language for Rails projects. Between the concise coding and enforced best practices, HAML continues to be a fun and efficient language to use.