Add Default Text for Text Inputs using jQuery
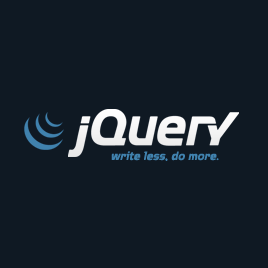
Adding a default text display in a text input is a common way to label a text input in order to provide instruction and clarity for a user. Here is a simple implementation using jQuery.
You can find the example here in jsFiddle.
First, let us add the HTML that will have a default text:
[code language=”html”]
<input type="text" name="username" default_text="Enter a username" />
[/code]
Here we added a default_text
attribute to the input. The default_text
will be used as the default text display. Next, let us code up the jQuery:
[code language=”javascript”]
$(‘input[default_text]’).bind(‘focus’, function() {
// Clear out default message.
if ($(this).hasClass(’empty’)) {
$(this).val(”);
$(this).removeClass(’empty’);
}
});
$(‘input[default_text]’).bind(‘blur’, function() {
// Add back the default message if the value is empty.
if ($(this).val() === ”) {
$(this).val($(this).attr(‘default_text’));
$(this).addClass(’empty’);
}
});
[/code]
Second, we have set up the event handlers for any input elements with a default_text
attribute. When the user focuses on a text input, the default text will clear. If the user blurs away from the text input, then the text input would re-populate with the default_text
value if the text value is empty. Here, we also add an empty
CSS class to mark if the input is empty.
Let’s initialize the default_text
inputs:
[code language=”javascript”]
$(‘input[default_text]’).each(function(index, el) {
$el = $(el);
$el.val($el.attr(‘default_text’));
$el.addClass(’empty’);
});
[/code]
Lastly, we have iterated through each default_text
input to mark it empty.
Note: You will need to pre-process the form data before submitting. We would not want to submit the default text. Here is an example how we can pre-process the form data:
[code language=”javascript”]
$(‘form#form_with_default_text’).bind(‘submit’, function() {
// Clear out default_text values if empty.
$(‘input[default_text].empty’).val(”);
});
[/code]
Last note: Currently, this implementation only works with input of type text
. This shouldn’t be used with an input of type password
. I’ll update this post later with a custom jQuery default text plugin that can handle both text and password types.