Android Custom Views: Creating an Animated Pie Chart from Scratch (Part 4 of 4)
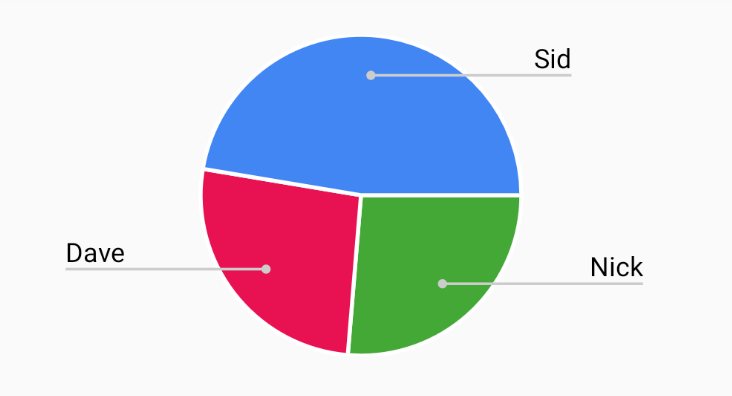
Introduction to Part 4
In Part 1, Part 2 , and Part 3 of this series, we covered the basics of custom views, created a simple custom PieChart class, and added state handling and custom methods to expand and collapse the pie chart in response to motion events.
In this fourth and final post, we’ll improve our state transitions with a bit of property animation.
Android property animation basics
Property animation refers to changing the value of a given property of an object over some period of time. The animated property can be just about anything: alpha, bounds, color, etc. The animated values are created by a ValueAnimator
object, which contains a listener for the values being generated.
The example below animates the alpha of a Paint
object, and highlights some of the ValueAnimator
methods we will be using:
A few notes on the above code:
- Line 4 creates a ValueAnimator of type
Int
that goes from 0 to 255 in value. - Line 5 specifies the amount of time that the animation should take to run (in this case, 400 milliseconds).
- Line 6 sets the interpolator, which dictates motion patterns for the animation; the
DecelerateInterpolator
slows the animation down over time. - Lines 7-10 add a listener to update the animated property as new values are generated by the
ValueAnimator
. Here, we change the green value of thePaint
object from 0 to 255, so that we transition smoothly between two different colors. Note that we also need to callinvalidate()
here, so that our view redraws with the new color. - Lines 11-16 add a new listener of type
AnimatorListenerAdapter
and override theonAnimationEnd()
function to set the color to yellow once the animation finishes (see AnimationListenerAdapter for a list of all functions that can be overridden in this way).
Adding animations to our pie chart
Let’s start by introducing a few ValueAnimator
s and two AnimatorSet
s. AnimatorSet is a class provided by Android that makes it very easy and seamless to trigger animations together or in sequence. AnAnimatorSet
is generally a good choice if you have animations in your app that make sense grouped together, or that need to fire at the same time.
Next, we’ll create a method to set the properties of all our animations. Let’s call it setupAnimations()
:
As you can see, we’re now setting the pieState
variable in an onAnimationEnd()
method, which is called when the animations complete. This is because we don’t want the user to be able to click between animations when the pieState
has already changed to the new state (this would cause the view to abruptly jump to the next animation sequence, which is not what we want).
We’ve also added two different types of interpolators for expanding and collapsing. This gives us a spring animation when we expand and a regular retreating pie chart as we collapse.
Lastly, we’ve set both of our AnimatorSet
s to play their respective animations.
The only thing left is to change our expandPieChart()
and collapsePieChart()
variables so they use their respective AnimatorSet
s:
All we’re doing here is setting the values for the ValueAnimator
s, and then calling start on the correct AnimatorSet
, which is already set up to play the animations in the correct order.
And there you have it — an animated pie chart!
[[@Sid: Can you insert the .gif here?]]