Analyzing Two Frontend Technologies: Vue vs. React
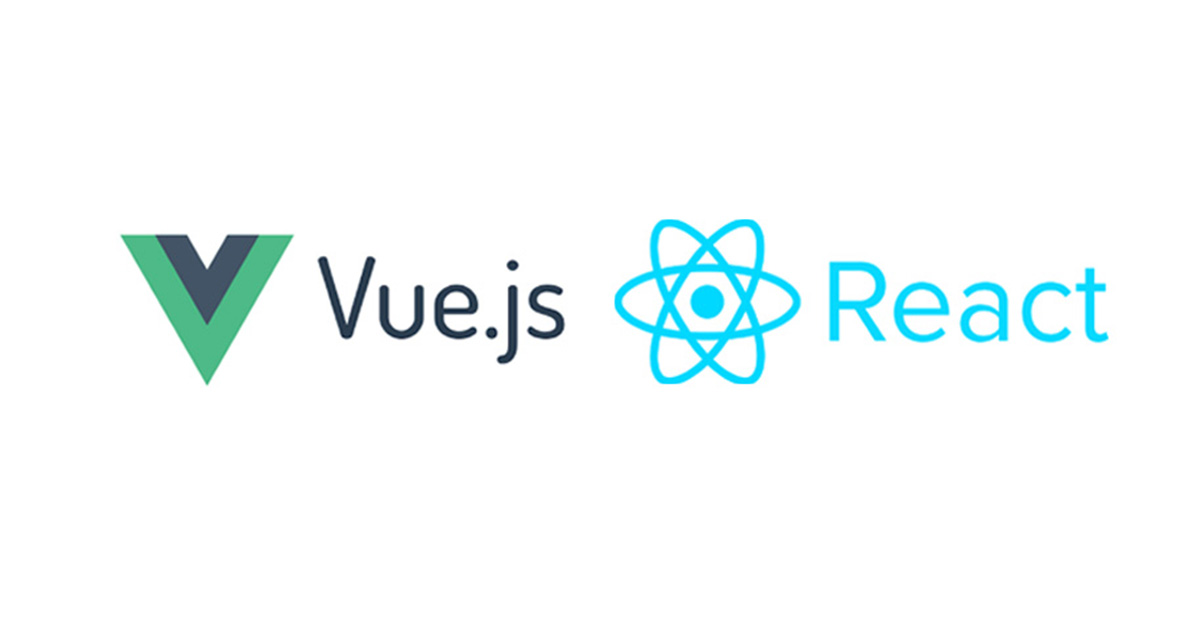
Two of the most popular front-end technologies today are React and Vue.js. Current usage statistics reveal that React has been the most-used front-end technology for quite some time, with none of the other options really challenging its position. However, Vue.js, a new, progressive JavaScript framework, is gaining popularity and boasting satisfaction ratings comparable to React’s.
This post provides my opinions on the strengths, weaknesses, and overall usability of these two frameworks. Though I’ve only spent about one year working with each, I’ve had the opportunity to explore many of the features they have to offer and see firsthand how each has been designed to respond to developer needs.
Vue.js
One of the defining features of Vue is its highly structured nature. Each file contains three parts: Template, Script, and Style. While only the Template is technically required, each of the three parts has its own unique responsibilities within the framework.
Template
Everything you know about HTML can be applied to the template in Vue. However, it also has “V-“ directives that allow you to inject logic into the template itself. For example:
- V-if: Allows you to show things conditionally.
- V-for: Loops through divisions of the template to repeat an action.
- V-bind: Connects variables to the component and triggers re-renders. If the bound variable changes, it will trigger a re-render so that the user can see an updated view.
- V-model: Provides two-way data binding and a nice shortcut for form values. The input the user gives is directly bound to a data attribute they can update directly.
- V-on: Allows for communication between parent and child components. Vue uses the emit model, in which the child component emits an action that the parent detects and responds to.
One of the template features that sets Vue apart is its slots feature. If you have a style that you want to apply to a child component, you can put the style in a slot, and the parent component can tell the child component what it’s going to display or load styles for it. This makes the interface much more customizable.
Script
The script is the location of all of your JavaScript and data manipulation. It is divided into different sections, each of which has its own responsibility:
- Data: Data is the variables that are local to that component.
- Computed: Computed is the section that gets the most kudos because it allows you to update values without taking much action. After setting assumptions for your data, whenever any of the values updates, your output value also updates, automatically forcing a re-render. Computed is a powerful action that React isn’t able to accomplish in the same way.
- Watch: Watch allows you to listen to the component data and run a function whenever that data changes.
- Methods: Methods is the location of all of your functions. Vue allows you to use a method wherever you would use a computed value.
- Props: Props can be passed from child to parent.
- Components: Components is one of the clunkier aspects of Vue. To use a component, you have to input the component and then it.
- Lifecycle: You can set data in the lifecycle to take care of the cleanup.
Style
Typically, you have a separate CSS file that will dictate what you want everything to look at, but with Vue, the style is defined at the bottom of the Vue file, so everything is all in one place and easy to find. This lends itself to a lot of customizability not present in other frameworks.
Style contains two components that make it especially customizable:
- Scoped: When defining your language within the style, you can define multiple style sections using scoped. Scoped ensures that the CSS will only apply the template to the specified files, allowing you to easily avoid style collisions.
- V-deep: The V-deep modifier allows you to tunnel in your styles so you can override styles to children components. This feature grants you additional flexibility within the style section.
Style also includes built-in animations, denoted as <Transitions />. Animations have certain keywords that automatically do things, rather than requiring you to input additional libraries.
Additional Vue Features
Since I have only used Vue for about a year, I haven’t had the opportunity to try out all the different features it offers. Some of the other features that people like in Vue include:
- Documentation: The Vue documentation is extensive and well-organized. The documentation explains every part of the Vue process in detail, along with caveats and examples. React documentation isn’t terrible, but it’s not on the level of Vue documentation.
- Built-in Features: Vue also comes in with a lot of built-in features, so you don’t have to rely on third parties for transitions (<transition />), routing (vue-router), or external state (Vuex).
- Learning the Language: The V-directives are part of Vue’s domain-specific language (DSL). There are a lot of things that you probably wouldn’t know just by using Vue; you’d have to go through the documentation or see someone else use it.
- Vue-cli: Also noted as a good thing for Vue, vue-cli provides the Vue command in your terminal and helps with new project creation.
React
React also contains many uniquely successful features. Though many of these features are more difficult to identify because the React documentation is not as extensive and each implementation of React is unique, some of the best features include:
- Everything is in JSX JavaScript Files: While Vue is in HTML, React allows you to get your HTML from the JavaScript.
- Functional Components: Functional components control React’s re-render cycle. React uses the idea of immutability, so React data isn’t changed; rather, a copy of the data is created and defined as a new state. When React detects a new state, the re-render is activated.
- Separate CSS: React typically has inline CSS with separate CSS files. My typical setup is an index file that defines all the JavaScript and then a CSS file that helps you style it.
- Hooks: Hooks are used to help you synchronize components and determine when to complete re-render cycles. There’s specifically a use-state hook that will trigger the whole re-render cascade.
- Callback Functions: In the React callback function, parent components pass the child component a function to use an action.
- Redux: Redux stores information for quick retrieval from the backend.
Same but Different
React and Vue both encounter similar problems in functionality. It can therefore be interesting to see how each framework resolves these problems in its own way:
Reusability
- Vue: Slots allow for customizable children with parents that have more control. Vue also uses name slots, which allows you to give each slot a unique name that the parent can use to tell the children exactly what custom content should go to each.
- React: React also uses slots, but does not have the same name slot content built in.
Re-Rendering Calculations
- Vue: Vue uses computed/watch to detect changes and re-render for the user. Computed is used for quick calculations that are cached, while watch then gives you more control over the re-render and the ability to induce side effects.
- React: React uses useMemo to cache results for quicker calculations and useEffect to induce side effects.
Re-Render Trigger
- Vue: If the values in the data section of the script are changed by mutations, it will trigger a re-render of that component or any of the children it was passed to.
- React: Immutable data is maintained by setState, so mutations do not occur. Instead, a new state is set, which triggers the render cascade.
Conditionals
- Vue: Domain-specific language (DSL) is used within the HTML itself to minimize wasted template space.
- React: Everything is in JavaScript, so it is fully customizable.
Children-Parent Communication
- Vue: The Emit Pattern allows children components to emit a specific name and data that can be caught by the parent unit.
- React: The parent passes the function down to the child.
Strengths
Though the size and overall performance of Vue and React are comparable, each framework has its own strengths:
Vue
- Documentation: Thorough, easy to use, easy to navigate documentation that is unmatched by other frameworks.
- Organization/Opinionatedness: You can always have three discrete sections (template, script, style) that each has a clearly defined responsibility. This lends itself to a much more opinionated way of doing code that standardizes how things get done. That can be good or bad depending on your preference.
- Easier Learning Curve: While there is a general consensus on this point, I don’t necessarily agree. I think if you’ve only done HTML, it’s easy to jump into Vue. However, getting used to the DSL and keywords is clunky.
- Built-in Features: The routing and state management features minimize the need for third parties.
- HTML-based: It is easy to transition projects from an HTML-base because you are able to carry over all of your CSS and HTML.
React
- Community support: According to the most recent polls, as many as 80% of developers use React, so it has a strong community support system.
- Third-Party Support: While React doesn’t have the built-in router and state management, there are third-party apps that are well-written and well-established.
- Lifecycle Control: The re-render timing can be manually controlled.
- JSX-based: Anything you can do in JavaScript you can do in React, giving you far more freedom than most frameworks.
Final Thoughts
My work with Vue over the last year has given me an appreciation for both its opinionatedness and clean file setup, while also making me incredibly aware of the learning curve. Though I am currently partial to Vue, both Vue and React are excellent frameworks worth exploring.