A Lightweight jQuery Notification Bar
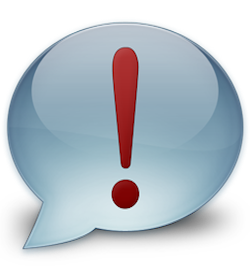
Notifying users with quick messages and alerts in a website is one of the most recurring needs of a web developer.
Every time I need to implement something like that, I start looking around the web hoping to find some Javascript/jQuery plugin that would do the work for me.
The good thing is that a lot of people have already faced this problem.
The bad thing is that the results of the search are way too many and often very confusing.
Chances are that most of the time, you don’t really need a 3MB-super-fancy-hyper-customizable-highly-priced plugin, but you just want something that works, and that you can quickly incorporate into your code.
If this is your case, feel free to use my 0MB-fancy-enough-minimally-customizable-chargeless notification bar.
Here’s a working demo:
And here’s the code:
[code lang=”javascript”]
function showNotificationBar(message, duration, bgColor, txtColor, height) {
/*set default values*/
duration = typeof duration !== ‘undefined’ ? duration : 1500;
bgColor = typeof bgColor !== ‘undefined’ ? bgColor : "#F4E0E1";
txtColor = typeof txtColor !== ‘undefined’ ? txtColor : "#A42732";
height = typeof height !== ‘undefined’ ? height : 40;
/*create the notification bar div if it doesn’t exist*/
if ($(‘#notification-bar’).size() == 0) {
var HTMLmessage = "<div class=’notification-message’ style=’text-align:center; line-height: " + height + "px;’> " + message + " </div>";
$(‘body’).prepend("<div id=’notification-bar’ style=’display:none; width:100%; height:" + height + "px; background-color: " + bgColor + "; position: fixed; z-index: 100; color: " + txtColor + ";border-bottom: 1px solid " + txtColor + ";’>" + HTMLmessage + "</div>");
}
/*animate the bar*/
$(‘#notification-bar’).slideDown(function() {
setTimeout(function() {
$(‘#notification-bar’).slideUp(function() {});
}, duration);
});
}
[/code]
(Available also on our Github: https://github.com/GrioSF/notificationBar)
Passing a set of parameters to the above function will allow you to customize the notification bar. Below you can find out what the parameters are meant for:
- message: the message you want to display in the bar (Default:””);
- bgColor: the bar background color (hex format) (Default: “#F4E0E1”);
- txtColor: the font color (hex format) (Default: “#A42732”);
- duration: how long will the bar be displayed for (milliseconds) (Default: 1500);
- height: the height of the bar (px) (Default: 40px);
The advantages of this notification bar are:
- No external plugins/.js files to include (assuming you already have jQuery);
- No external css/images needed;
- less than 20 lines of code to copy paste;
- free and editable;
Typical usages of the bar could be:
- error message when submitting a form or a login
- successful updates messages
- welcome message
- error messages alerting of a wrong click
- instruction messages
- debug messages
What are you waiting for? Use the code and have fun notifying your users!
Suggestions and feedback are as always welcome in the comments section.
4 Comments