Meet Kotlin
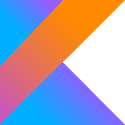
Kotlin is a JVM language that hit version 1.0 about a year ago (February 2016).
It is developed by JetBrains, the same people who make my favorite suite of
IDEs. The language itself is open-source under the Apache License 2.0 and is
developed as a community project over at kotlinlang.org. Kotlin is something
that I have become rather excited about over the past year. This post’s goal is
not to teach you Kotlin but to get you excited about it!
Goals of Kotlin
The stated goals of Kotlin are: Interoperability, safety, and tooling.
Interoperability
Kotlin is designed to be 100% interoperable with Java. There is always the
ability to use Java libraries from Kotlin, just as there is the option to use
Kotlin libraries from Java. It is even extremely easy to mix Java and kotlin
within the same codebase without a problem. See the documentation for
more info regarding interoperability
Safety
Type safety is important to the design of Kotlin as well. It makes an attempt to
catch type errors at compile time in order to avoid runtime instability.
Tooling
While Scala has many of the features that the Kotlin team wanted in a language,
it took significantly longer to compile and had significant runtime
dependencies. Kotlin has a stated goal of being as fast to compile as Java and
does not have a runtime dependency (though it does need its standard library,
I think.)
There are official Kotlin plugins/support for IntelliJ IDEA (community and
ultimate), Android Studio, Gradle, Maven, Ant, even Eclipse.
Syntax
In Kotlin the type comes after the name. If you use Typescript, Go, Swift, etc
you’ll be familiar with this concept.
var city : String = "San Francisco" | |
val population : Int = 864816 // 2015 data from wikipedia |
Additionally, var
is non-final, but a val
is final
Functions are not required to belong to a class, and are defined with a fun
keyword.
fun greet(name : String) : String { | |
println("Hello, ${name}") | |
} |
Where can I use Kotlin?
You can use Kotlin (nearly) anywhere you are already using Java. It can be added
into a backend project relying on Spring or Play frameworks with ease. It is
also gaining huge popularity in the Android development community.
Kotlin on Android is what drew me to the language in the first place. I, along
with much of the Android development community, had a growing desire for a less
verbose, more concise, more modern language to build our applications with. The
fact that Android Studio fully supports Kotlin out of the box is a huge help to
knocking down the barriers of adding Kotlin into an existing codebase, as well
as making it easy to start new projects with Kotlin.
Language Features
Now for the fun part: showing off the features of Kotlin. My favorite way to
introduce Kotlin is not to answer “what can I do with Kotlin?”, but instead
answer “what don’t I need to do in Kotlin?”.
What don’t you need to do in Kotlin?
Pretend you need to make a lightweight model class to represent a person. For
simplicity’s sake, a person can have a name, age, and a city. You want to be
able to compare two person objects (equals()
and hashCode
) as well as print
out the model for logging or debugging reasons (toString()
).
In Java this lightweight model looks something like this:
public class Person { | |
public final String name; | |
public final int age; | |
public final String city; | |
public Person(String name, int age, String city) { | |
this.name = name; | |
this.age = age; | |
this.city = city; | |
} | |
@Override | |
public boolean equals(Object o) { | |
if (this == o) return true; | |
if (o == null || getClass() != o.getClass()) return false; | |
Person person = (Person) o; | |
if (age != person.age) return false; | |
if (name != null ? !name.equals(person.name) : person.name != null) return false; | |
return city != null ? city.equals(person.city) : person.city == null; | |
} | |
@Override | |
public int hashCode() { | |
int result = name != null ? name.hashCode() : 0; | |
result = 31 * result + age; | |
result = 31 * result + (city != null ? city.hashCode() : 0); | |
return result; | |
} | |
@Override | |
public String toString() { | |
return "Person{" + | |
"name='" + name + '\'' + | |
", age=" + age + | |
", city='" + city + '\'' + | |
'}'; | |
} | |
} |
We have 40 lines of code to achieve this simple and common task. Kotlin can do
all of this using its data class
functionality… in one line.
data class Person(val name: String, val age: Int, val city: String) |
A data class has final fields defined after the class name, it generates
(internally) the constructor, equals()/hashCode()
, toString()
, copy()
(not used in my example), and componentN()
functions
Safety
A value must be assigned either at the time of declaration or during instance
creation. If not, it will throw a compiler error
var a = 5 // fine | |
var b : Int // error, needs a value |
To allow for a value to potentially be null, you must use the ?
operator on
the type. If you have a potentially null type, you must safe-call (?.
) or
assert that you know more than the compiler (!!.
)
var a : String? = null | |
println(a.length) // error | |
println(a?.length) // prints "null" since a?.length is null since a is null | |
println(a!!.length) // compiles, crashes with NPE |
Other nifty features
Destructuring
var (a, b) = listOf(1, 2) |
Single value functions
fun year() = 2017 |
Lambdas and iterators
// double each value | |
listOf(1, 2, 3, 4, 5).forEach { it * 2 } | |
// Only even values (use a named iterator param, rather than the default 'it') | |
listOf(1, 2, 3, 4, 5).filter { v -> v % 2 == 0 } | |
// You know you'll be filtering out nulls alot... | |
listOf(1, 2, null, 4).filterNotNull() |
Extensions
To me, this is quite similar to “monkey-patching” in Ruby. Officially, it draws
inspiration from C# and Gosu.
Here is a simple example of adding a .reverse()
method to String
s.
fun String.reverse() : String { | |
var r = "" | |
forEach { r = it + r } | |
return r | |
} | |
// usage: | |
"hello world".reverse() |
There is so much more…
This is hardly an exhaustive showcase of Kotlin’s features. I hope that it was
able to get you excited or thinking about trying something new on a project
though! If you’d like a relatively safe way to get started, try dropping kotlin
into an existing project and using it to write some tests. Your tests will
become shorter, more clear, and you’ll hopefully want to use Kotlin everywhere!
I’m intending to use Kotlin any time I have the opportunity. I have high hopes
for this young language that shows lots of promise, hopefully you’ll join in on the
excitement!
1 Comment